Rollover (Mouseover) is one of the most simplest and at the same time the most popular script on web-pages. Nevertheless in 90% of the cases this simple script is made incorrectly. The fact!
We first need to understand how this script should work. At first sight it looks quite elementary: Whenever the user moves the mouse pointer over the button, the button changes own state (one picture is replaced with another).
It should appear immediately but often it does not show. Why? The fact is that the second(active) picture downloads only WHEN user moves the mouse pointer over the button. Even if the user have hi-speed Internet connection - it will take away some time for that. The more the size of the picture - the more delay.
Sample of incorrect rollover button
When you move the mouse pointer over the button for the first time you may see a small lag: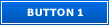
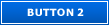
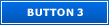
Preload image
You need to use this script for load images as soon as possible. Insert this code between your <head></head> tags. Edit this script (see the comments).<script type="text/javascript">
<!--
if(document.images)
{
var image_array = new Array();
// path to the directory with images
var path = '/img/';
// enumeration of the "active" images
image_array[0] = path + "button1_red.gif";
image_array[1] = path + "button2_red.gif";
image_array[2] = path + "button3_red.gif";
var preload_image = new Array ();
for(var i=0; i<image_array.length; i++)
{
preload_image[i]= new Image();
preload_image[i].src = image_array[i];
}
}
//-->
</script>
After the images were loaded - we use usual rollover buttons html code.HTML code
<a href="#" onmouseover="rollover('button1','button1_red.gif')" onmouseout="rollover('button1','button1_blue.gif')">
<img src="/img/button1_blue.gif" name="button1" width="109" height="25" border="0"></a>
<a href="#" onmouseover="rollover('button2','button2_red.gif')" onmouseout="rollover('button2','button2_blue.gif')">
<img src="/img/button2_blue.gif" name="button2" width="109" height="25" border="0"></a>
<a href="#" onmouseover="rollover('button3','button3_red.gif')" onmouseout="rollover('button3','button3_blue.gif')">
<img src="/img/button3_blue.gif" name="button3" width="109" height="25" border="0"></a>
The onmouseover and onmouseout event handlers is used to execute specified Javascript code whenever the user moves the mouse over an button or move out from button.
Rollover function
Insert this code into any place of yours html, but BEFORE a html-code of buttons:<script type="text/javascript">
<!--
function rollover(name, filename)
{
var fullpath = '/img/' + filename;
document.images[name].src = fullpath;
}
//-->
</script>
Correct sample
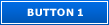
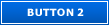
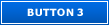
Note: Set fullpath variable to ="" if the images placed in the same directory as the html-file.